Giới thiệu
Giao thức truyền tệp an toàn (SFTP) là một trong những cách tiếp cận để tải lên máy chủ từ xa qua kết nối an toàn và được mã hóa. Trong .NET, chúng ta có thể dễ dàng phát triển một tiện ích để thực hiện tác vụ đã đề cập.
Đối với điều này, tất cả những gì chúng ta cần làm là sử dụng một assembly có tên là SSH.NET. SSH.NET là một thư viện Secure Shell (SSH) cho.NET, được tối ưu hóa cho song song và hỗ trợ khuôn khổ rộng.
Chúng ta có thể cài đặt thư viện này bằng cách thực hiện lệnh sau trong bảng điều khiển trình quản lý gói theo các bước sau
1. Click phải vào dự án muốn thêm chọn Manage NuGet Package...
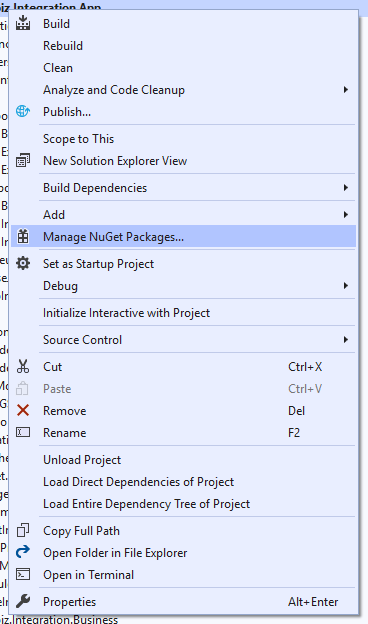
2. Nhập SSH và click install như hình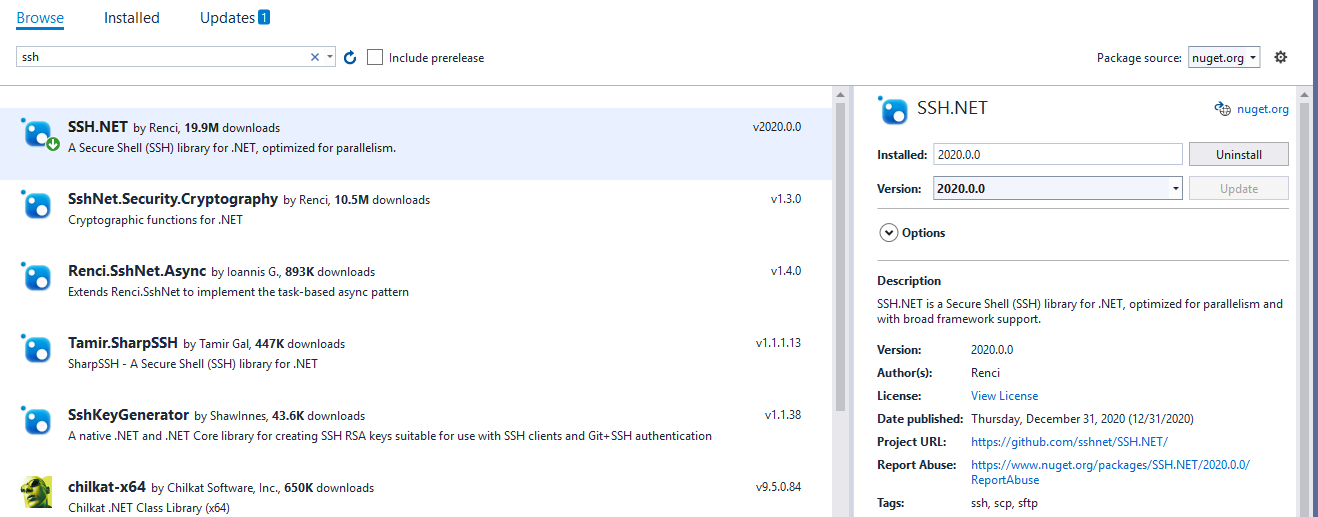
Vậy là bạn đã cài đặt thành công gói package SSH.NET
Bây giờ code thôi. Bài toán đặt ra là mình sẽ up một file lên server. Server này có các thông tin như ip, port, username, pass.
Và mình sẽ làm như sau:
1. Check tồn tại folder
2. Tạo folder
3. Check tồn tại file
4. Xóa file
5. Upload file từ local lên ftp
Trong hàm xử lý chính của mình sẽ như sau:
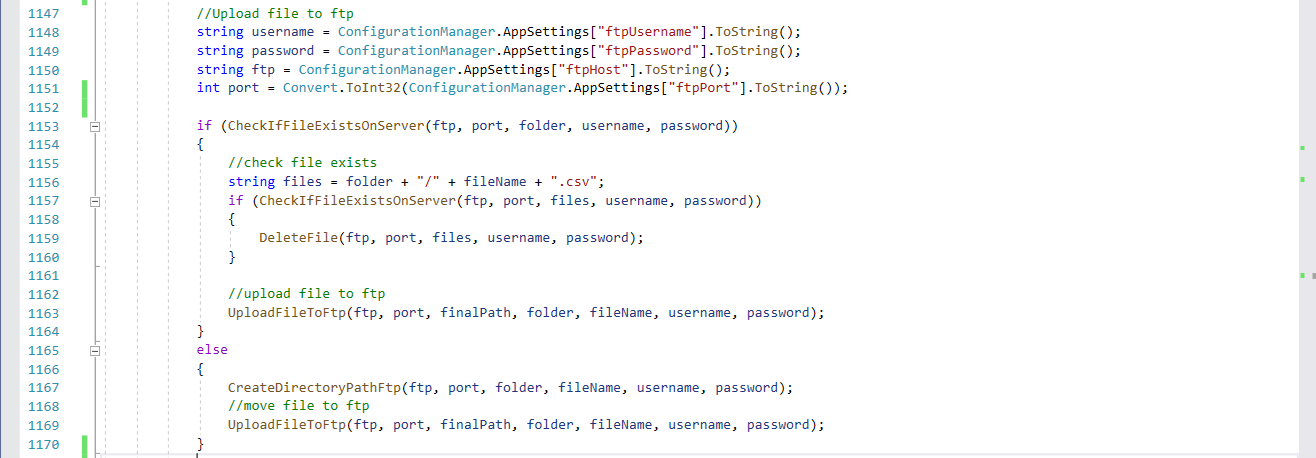
Các thông tin về username, pass, ip, port mình sẽ để trong config và lấy ra như trên.
Code detail:
string username = ConfigurationManager.AppSettings["ftpUsername"].ToString();
string password = ConfigurationManager.AppSettings["ftpPassword"].ToString();
string ftp = ConfigurationManager.AppSettings["ftpHost"].ToString();
int port = Convert.ToInt32(ConfigurationManager.AppSettings["ftpPort"].ToString());
if (CheckIfFileExistsOnServer(ftp, port, folder, username, password))
{
//check file exists
string files = folder + "/" + fileName + ".csv";
if (CheckIfFileExistsOnServer(ftp, port, files, username, password))
{
DeleteFile(ftp, port, files, username, password);
}
//upload file to ftp
UploadFileToFtp(ftp, port, finalPath, folder, fileName, username, password);
}
else
{
CreateDirectoryPathFtp(ftp, port, folder, fileName, username, password);
//move file to ftp
UploadFileToFtp(ftp, port, finalPath, folder, fileName, username, password);
}
Hàm xử lý kiểm tra folder, file tồn tại
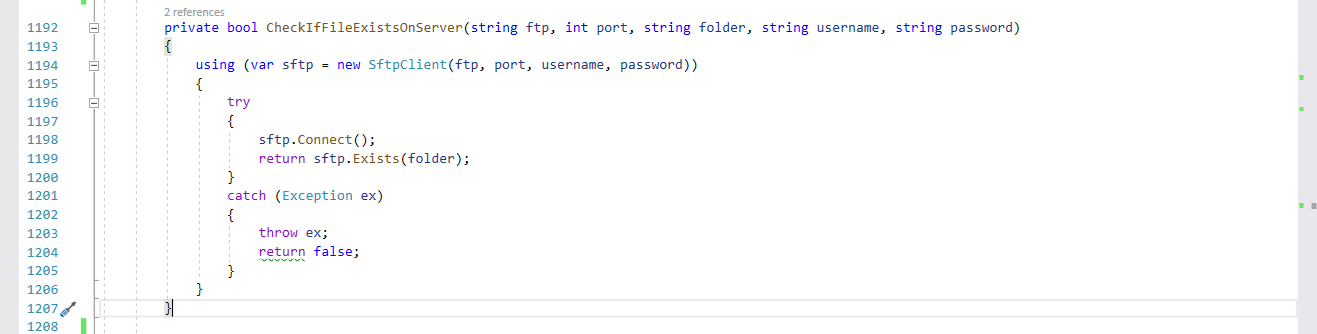
Code Detail:
private bool CheckIfFileExistsOnServer(string ftp, int port, string folder, string username, string password)
{
using (var sftp = new SftpClient(ftp, port, username, password))
{
try
{
sftp.Connect();
return sftp.Exists(folder);
}
catch (Exception ex)
{
throw ex;
return false;
}
}
}
Hàm xóa files, folder
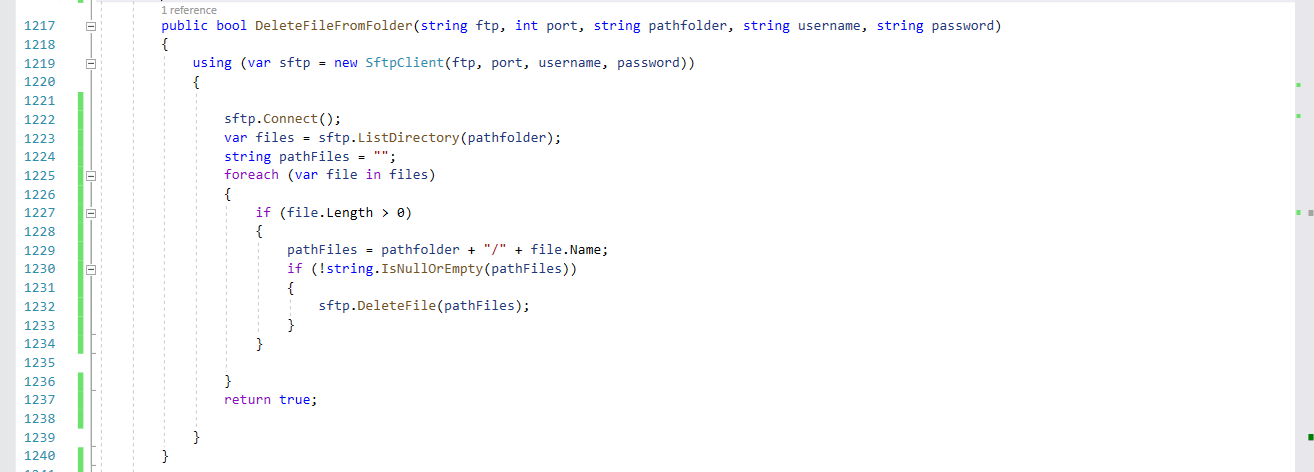
Code Detail:
public bool DeleteFileFromFolder(string ftp, int port, string pathfolder, string username, string password)
{
using (var sftp = new SftpClient(ftp, port, username, password))
{
sftp.Connect();
var files = sftp.ListDirectory(pathfolder);
string pathFiles = "";
foreach (var file in files)
{
if (file.Length > 0)
{
pathFiles = pathfolder + "/" + file.Name;
if (!string.IsNullOrEmpty(pathFiles))
{
sftp.DeleteFile(pathFiles);
}
}
}
return true;
}
}
Hàm Upload file lên server ftp
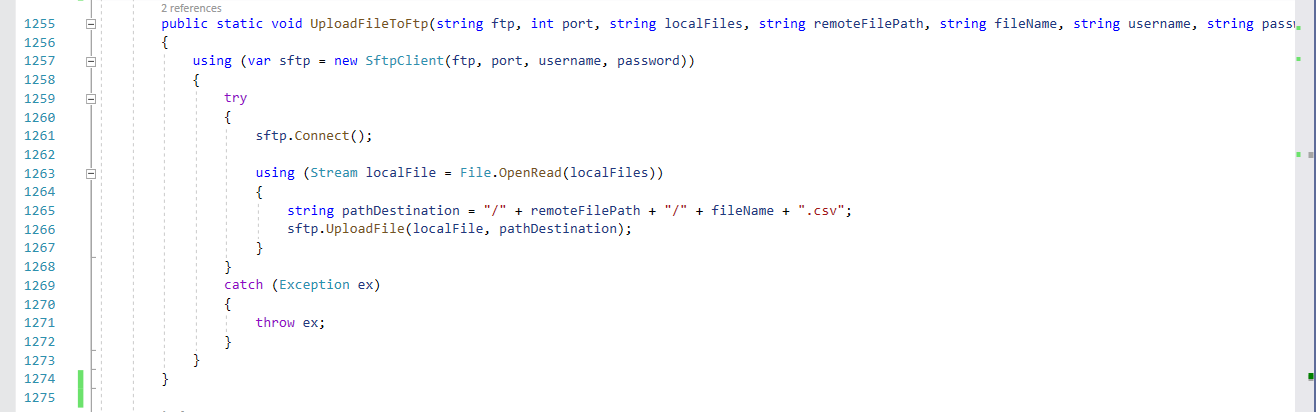
Code Detail:
public static void UploadFileToFtp(string ftp, int port, string localFiles, string remoteFilePath, string fileName, string username, string password)
{
using (var sftp = new SftpClient(ftp, port, username, password))
{
try
{
sftp.Connect();
using (Stream localFile = File.OpenRead(localFiles))
{
string pathDestination = "/" + remoteFilePath + "/" + fileName + ".csv";
sftp.UploadFile(localFile, pathDestination);
}
}
catch (Exception ex)
{
throw ex;
}
}
}
Hàm download file từ ftp
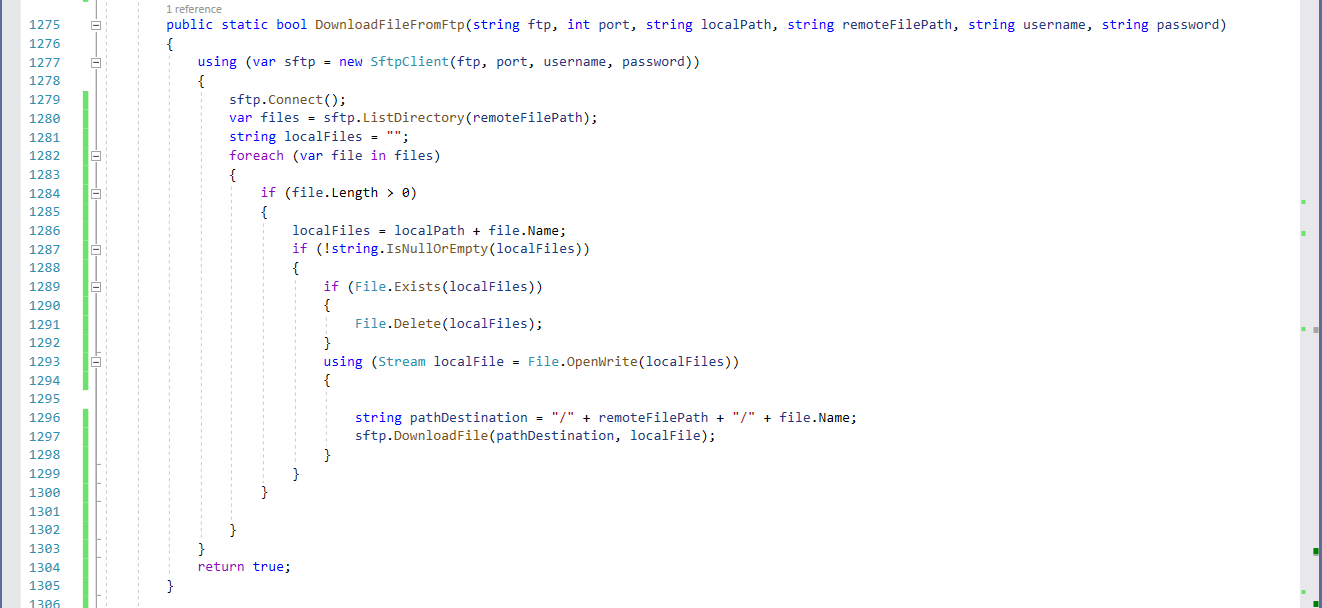
Code Detail:
public static bool DownloadFileFromFtp(string ftp, int port, string localPath, string remoteFilePath, string username, string password)
{
using (var sftp = new SftpClient(ftp, port, username, password))
{
sftp.Connect();
var files = sftp.ListDirectory(remoteFilePath);
string localFiles = "";
foreach (var file in files)
{
if (file.Length > 0)
{
localFiles = localPath + file.Name;
if (!string.IsNullOrEmpty(localFiles))
{
if (File.Exists(localFiles))
{
File.Delete(localFiles);
}
using (Stream localFile = File.OpenWrite(localFiles))
{
string pathDestination = "/" + remoteFilePath + "/" + file.Name;
sftp.DownloadFile(pathDestination, localFile);
}
}
}
}
}
return true;
}
Hàm tạo path folder trên server ftp
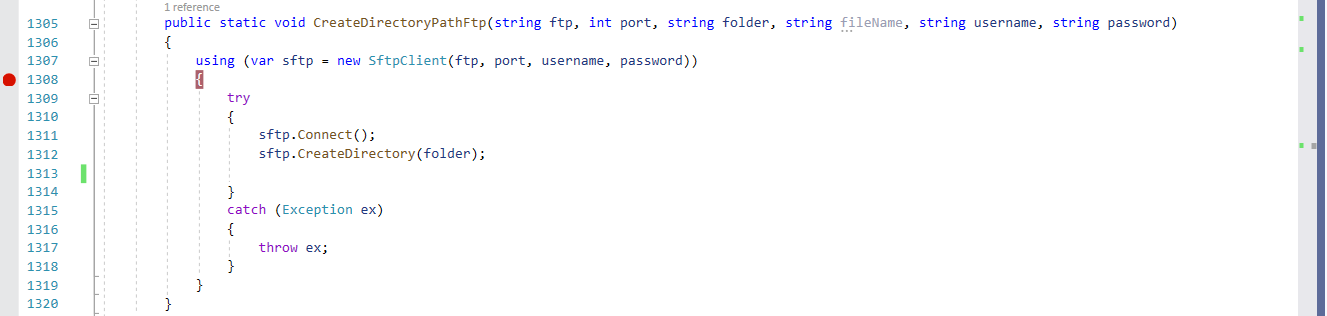
Code Detail:
public static void CreateDirectoryPathFtp(string ftp, int port, string folder, string fileName, string username, string password)
{
using (var sftp = new SftpClient(ftp, port, username, password))
{
try
{
sftp.Connect();
sftp.CreateDirectory(folder);
}
catch (Exception ex)
{
throw ex;
}
}
}
Hy vọng qua bài viết này sẽ giúp các bạn có thể thao tác check tồn tại, download file, upload file. Cảm ơn các bạn theo dõi.
Bài viết liên quan:
- Cách import data csv vào sql server sử dụng c# (Import csv to sql server c#)
- Cách xuất file csv từ list object sử dụng c# (Export list object to csv c#)